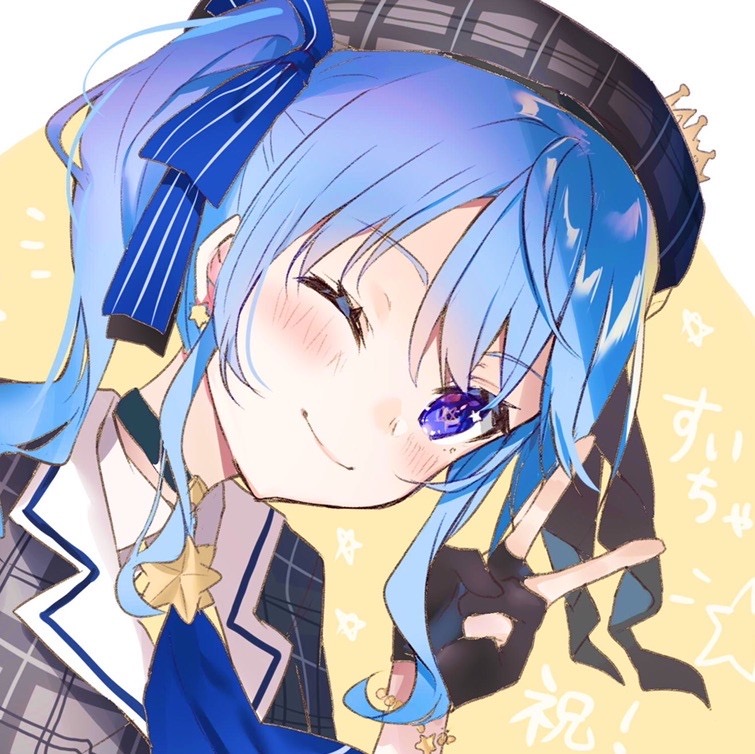
本系列的博客的内容是LLVM异常实现的整个过程,从C++生成LLVM IR开始,到运行时实际调用的库函数,会从抛出异常的过程开始结合llvm相关的代码进行讲解。这一期主要内容是讲解异常相关的结构、底层实现方式等基础信息,之后从顶向下逐层分解其中的实现(这部分形式有些类似于笔记),在最后一期会将整个结构串起来,同时有一个流程图供读者进行参考,中间几期细节比较多,很容易迷失在其中,可以参照最后一期的图来阅读中间的内容。
多层结构
先说结论,异常主要由两部分组成
- 语言相关的abi实现
- 语言无关的部分(调用libunwind库)
其中语言相关的abi实现需要传递信息给libunwind,比如说一些情况要怎么处理,传递符合要求的文件头等
语言相关的实现
当我们编写编程语言的时候,不同的语言有不同的异常语法。比如说常见的对于一个块做try,捕获产生的不同Exception。假设这些语言都接入llvm进行代码生成,尽管编程语言有着不同的语法,但在用语法树生成llvm代码时都会生成类似的内容。
以下用C++举例,这是一段C++代码
1 | void f1() |
执行clang++ -S -emit-llvm main.cpp && cat main.ll查看对应的llvm ir
1 | @.str = private unnamed_addr constant [6 x i8] c"error\00", align 1 |
我们来看编译出的三个函数,对于未throw的f1来说,相比f2多了一个nounwind这一个attr,并且多了两个函数调用。而调用了f1和f2的f3,因为调用了f2这个需要unwind的函数因此和f2同样没有nounwind的attr。
关于这个attr含义也很简单,用于标明函数是否会抛出异常。以下是LLVM reference中的原始文档
This function attribute indicates that the function never raises an exception. If the function does raise an exception, its runtime behavior is undefined. However, functions marked nounwind may still trap or generate asynchronous exceptions. Exception handling schemes that are recognized by LLVM to handle asynchronous exceptions, such as SEH, will still provide their implementation defined semantics.
接着我们来看两个令人在意的函数调用:__cxa_allocate_exception和 __cxa_throw
这些是在libcxxabi中的函数,看名字我们能大概猜到其中的含义,一个是分配exception另一个则是抛出。
我们在这里先简单窥探一下__cxa_throw的实现
1 | void |
可以看到其中调用了_Unwind_RaiseException,这个函数是属于libunwind库的一个接口,而libunwind中则再无其他库的引用,这印证了前面提到的异常实现的两部分:语言相关的abi和libunwind。
libunwind的实现
libunwind中主流的异常实现方式有三类
- seh(structure exception handling),在Windows上使用,但官方建议使用ISO标准C++异常处理
- ehabi(exception handling application binary interface),arm中定义的二进制接口,定义如何传递和处理异常的规则。
- sjlj(setjmp / longjmp),通过jmp指令跳转到异常处理代码。同时ehframe和dwaf经常与sjlj联合使用
https://learn.microsoft.com/zh-cn/cpp/cpp/structured-exception-handling-c-cpp?view=msvc-170
- 本文标题:LLVM异常实现零 异常的多层结构与实现方式
- 本文作者:Homura
- 创建时间:2024-10-02 11:27:36
- 本文链接:https://homura.live/2024/10/02/Exception/exception-0/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!